Rsa Algorithm For Key Generation And Cipher Verification In Java
- I know how to generate rsa keys and encrypt an decrypt. My question what the difference is between a Java generated rsa key and a rsa key generated with openssh. Is there a way to convert this key in java? – Ralph Oct 23 '13 at 6:33.
- Dec 28, 2016 Example of RSA generation, sign, verify, encryption, decryption and keystores in Java - RsaExample.java.
- RSA Encryption Javascript and Decrypt Java. Spent almost 2 days with different combinations.I am generating a asymmetric key pair (public and private) in java using RSA algorithm and trying to use the public key in javascript to encrypt some text and decrypt back in java on server side.
How to Encrypt Decrypt File in Java? Using Cipher class and RSA algorithm we can encrypt and decrypt a file. What is Cipher Class? This is a java class, use cryptographic algorithm for encryption and decryption. RSA Algorithm. RSA, is an asymmetric cryptographic algorithm used for message encryption. ∟ RsaKeyGenerator.java for RSA Key Generation This section describes the initial draft of a RSA public key and private key generation implementation using the java.math.BigInteger class. The first step of RSA public key encryption implementation is to write a program to generate a pair of public key and private key.
importjavax.crypto.Cipher; |
importjava.io.InputStream; |
importjava.security.*; |
importjava.util.Base64; |
import staticjava.nio.charset.StandardCharsets.UTF_8; |
publicclassRsaExample { |
publicstaticKeyPairgenerateKeyPair() throwsException { |
KeyPairGenerator generator =KeyPairGenerator.getInstance('RSA'); |
generator.initialize(2048, newSecureRandom()); |
KeyPair pair = generator.generateKeyPair(); |
return pair; |
} |
publicstaticKeyPairgetKeyPairFromKeyStore() throwsException { |
//Generated with: |
// keytool -genkeypair -alias mykey -storepass s3cr3t -keypass s3cr3t -keyalg RSA -keystore keystore.jks |
InputStream ins =RsaExample.class.getResourceAsStream('/keystore.jks'); |
KeyStore keyStore =KeyStore.getInstance('JCEKS'); |
keyStore.load(ins, 's3cr3t'.toCharArray()); //Keystore password |
KeyStore.PasswordProtection keyPassword =//Key password |
newKeyStore.PasswordProtection('s3cr3t'.toCharArray()); |
KeyStore.PrivateKeyEntry privateKeyEntry = (KeyStore.PrivateKeyEntry) keyStore.getEntry('mykey', keyPassword); |
java.security.cert.Certificate cert = keyStore.getCertificate('mykey'); |
PublicKey publicKey = cert.getPublicKey(); |
PrivateKey privateKey = privateKeyEntry.getPrivateKey(); |
returnnewKeyPair(publicKey, privateKey); |
} |
publicstaticStringencrypt(StringplainText, PublicKeypublicKey) throwsException { |
Cipher encryptCipher =Cipher.getInstance('RSA'); |
encryptCipher.init(Cipher.ENCRYPT_MODE, publicKey); |
byte[] cipherText = encryptCipher.doFinal(plainText.getBytes(UTF_8)); |
returnBase64.getEncoder().encodeToString(cipherText); |
} |
publicstaticStringdecrypt(StringcipherText, PrivateKeyprivateKey) throwsException { |
byte[] bytes =Base64.getDecoder().decode(cipherText); |
Cipher decriptCipher =Cipher.getInstance('RSA'); |
decriptCipher.init(Cipher.DECRYPT_MODE, privateKey); |
returnnewString(decriptCipher.doFinal(bytes), UTF_8); |
} |
publicstaticStringsign(StringplainText, PrivateKeyprivateKey) throwsException { |
Signature privateSignature =Signature.getInstance('SHA256withRSA'); |
privateSignature.initSign(privateKey); |
privateSignature.update(plainText.getBytes(UTF_8)); |
byte[] signature = privateSignature.sign(); |
returnBase64.getEncoder().encodeToString(signature); |
} |
publicstaticbooleanverify(StringplainText, Stringsignature, PublicKeypublicKey) throwsException { |
Signature publicSignature =Signature.getInstance('SHA256withRSA'); |
publicSignature.initVerify(publicKey); |
publicSignature.update(plainText.getBytes(UTF_8)); |
byte[] signatureBytes =Base64.getDecoder().decode(signature); |
return publicSignature.verify(signatureBytes); |
} |
publicstaticvoidmain(String.. argv) throwsException { |
//First generate a public/private key pair |
KeyPair pair = generateKeyPair(); |
//KeyPair pair = getKeyPairFromKeyStore(); |
//Our secret message |
String message ='the answer to life the universe and everything'; |
//Encrypt the message |
String cipherText = encrypt(message, pair.getPublic()); |
//Now decrypt it |
String decipheredMessage = decrypt(cipherText, pair.getPrivate()); |
System.out.println(decipheredMessage); |
//Let's sign our message |
String signature = sign('foobar', pair.getPrivate()); |
//Let's check the signature |
boolean isCorrect = verify('foobar', signature, pair.getPublic()); |
System.out.println('Signature correct: '+ isCorrect); |
} |
} |
commented Oct 17, 2019
It's good thank you so much , How can i create base64 like jwt (header,body,sign) ? |
commented Nov 26, 2019
Thanks for the code. Adobe premiere pro cs6 family key generator. One issue - using |
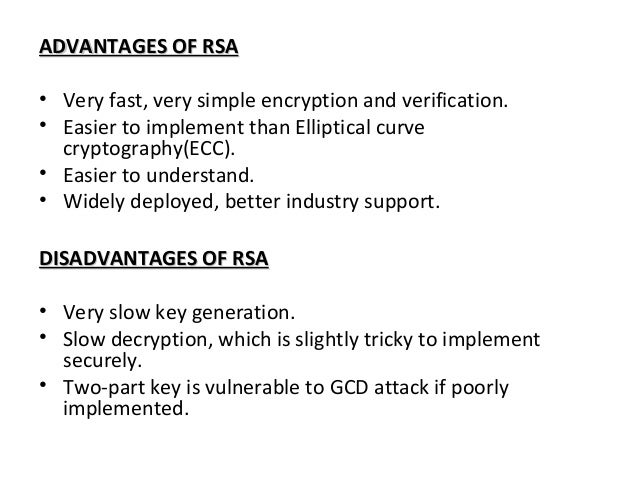
Rsa Algorithm For Key Generation And Cipher Verification In Java Code
commented Dec 29, 2019
@stdunbar: It depends on your keyStore creation. Php generate public private key pair. |